Exploring Python Byte Code - Compilers Vs. Interpreters
Discover the key differences between compilers and interpreters in programming. Learn how each approach processes code, their advantages, and their role in modern software development, including a deep dive into Python's hybrid approach.
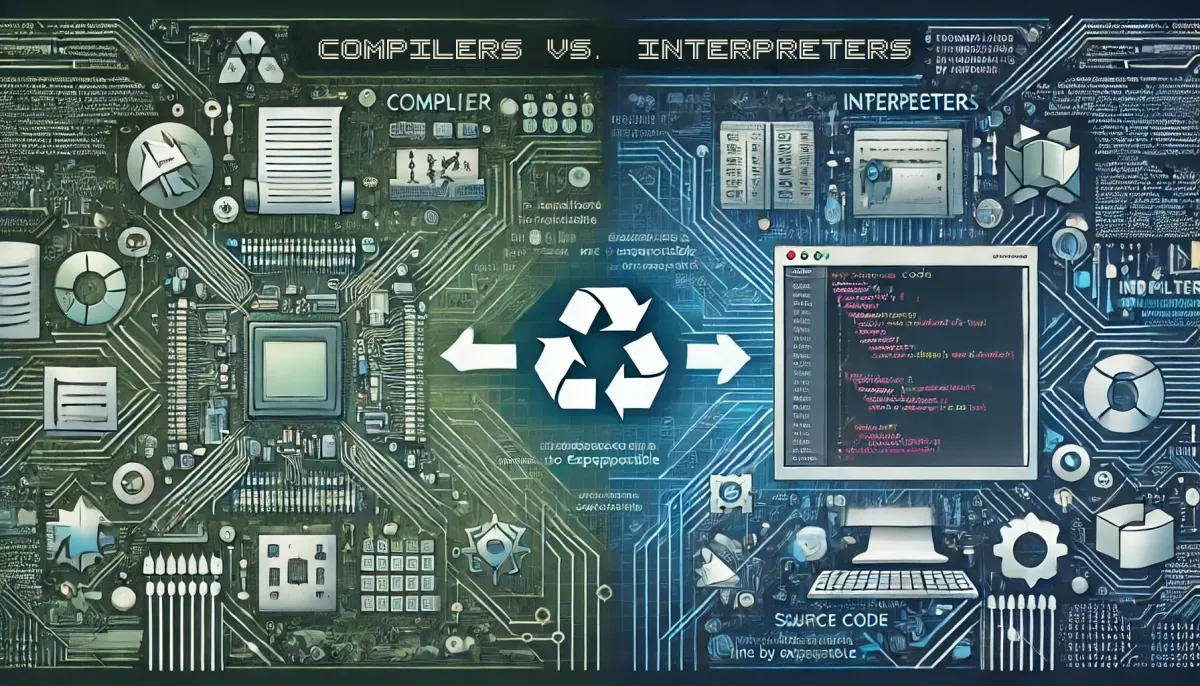
Introduction
Before we dive into the intricacies of Python byte code, let's first understand the different approaches programming languages use to execute the programs we write.
As we know, computers only understand a limited set of instructions; even those instructions are just collections of bytes. But humans would have a hard time expressing solutions to problems or applications directly a streams of ones and zeroes.
This is why programming languages were invented. However, before anything written in a programming language is executed, it has to be encoded into a format a computer can understand.
This first article will discuss some of the approaches programming languages can take to move from source code to execution: interpreting, compiling, and transpiling.
Compilers vs. Interpreters
The two principal approaches to executing programs are interpreting and compiling them.
Let's discuss the fundamental differences between compilers and interpreters.
Compilers
A compiler translates a program's entire source code from a high-level programming language into machine code, which the computer’s processor can directly execute. This translation happens before the program runs, resulting in a separate executable file.
In some sense, a compiler is similar to a person who translates books or documents from one language to another. For example, Gregory Rabassa translated "Cien años de soledad" into "One hundred years of solitude."
Here are some critical characteristics of compilers:
- Translation: Compilers translate the entire program in one go, creating an executable file. The executable file is specific to an Operating System and machine architecture, which is part of the reason why we can't execute a Mac application on a Linux machine or vice versa.
- Speed: The executable runs quickly once compiled since it's already in machine code.
- Error Detection: Compilers detect errors during the compilation process. If there are errors, the executable is not created.
- Optimization: Because compilers process the entire program, they can optimize code during the translation process to improve performance.
Examples of Compiled Languages:
Interpreters
Conversely, an interpreter translates source code into machine code on the fly, executing the code line by line as it runs. This means there is no separate executable file; the source code is executed directly.
An interpreter in the UN is a person who simultaneously translates speeches into a different language. This is what a programming language interpreter does; it translates on the fly, does not know what will come next, and might only maintain a limited memory of what was said before.
An interpreter doesn't analyze the complete program. This limits the opportunities for optimizations and error detection in exchange for flexibility and ease of implementation.
Here are some critical characteristics of interpreters:
- Translation: Interpreters translate and execute code line by line.
- Speed: Interpreted programs can be slower since translation happens at runtime.
- Error Detection: Errors are detected at runtime, which can make debugging easier since you see the error in the context of the execution.
- Flexibility: Interpreters offer more flexibility and are often used in scripting languages where rapid development and execution are needed.
Examples of Interpreted Languages:
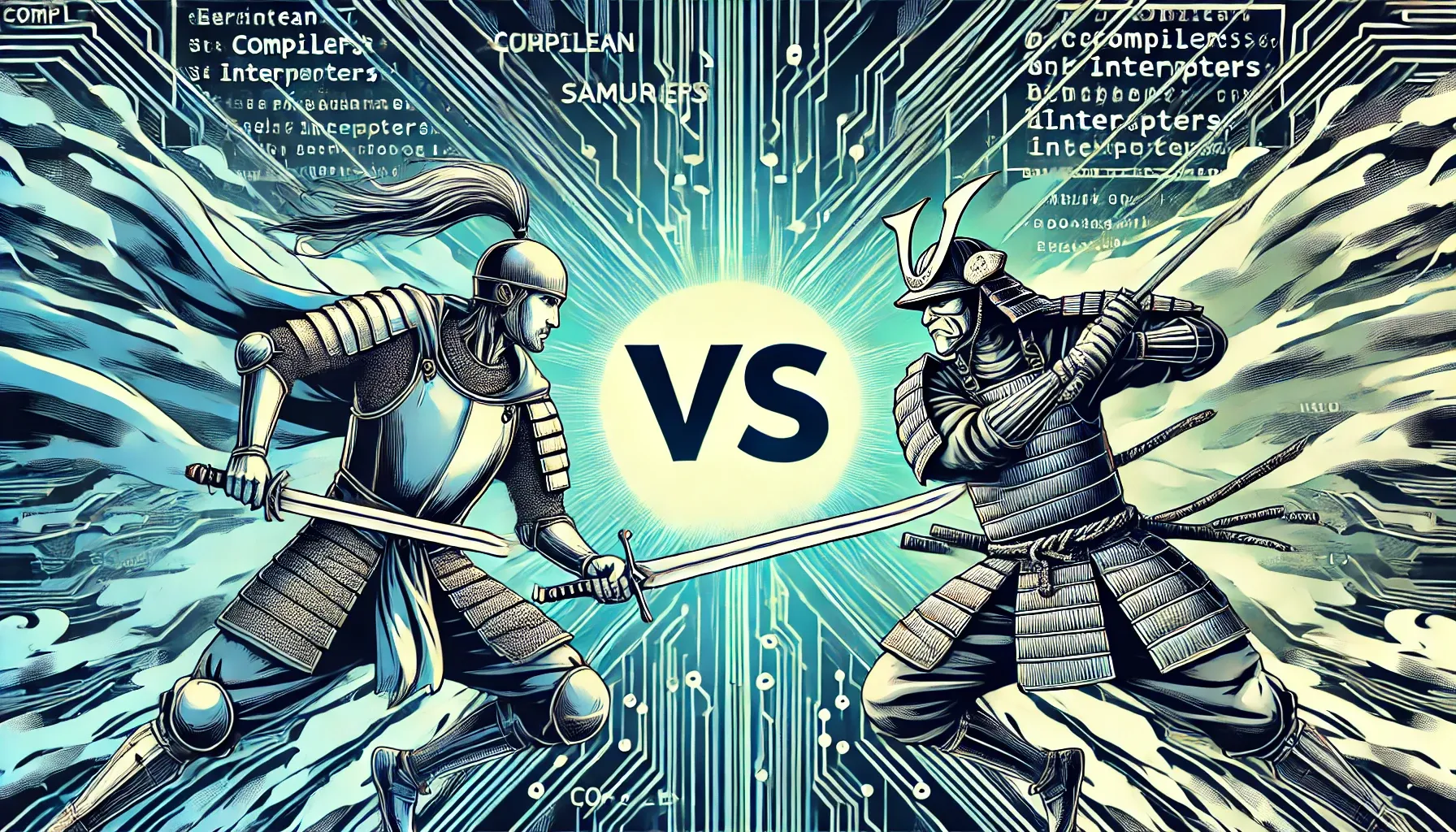
Java and JVM Languages: A Hybrid Approach
Java and other languages that run on the Java Virtual Machine (JVM), such as Kotlin and Scala, use a hybrid approach. Here's how it works:
- Compilation to Byte Code: The source code is compiled into an intermediate form called byte code, which is not directly executable by the computer's processor but by the JVM.
- Execution by the JVM: The JVM interprets the byte code and may also compile it into machine code at runtime using Just-In-Time (JIT) compilation for better performance.
This approach combines the benefits of both compilation and interpretation:
- Portability: Byte code can be executed on any platform that has the JVM, making Java programs highly portable.
- Efficiency: The JVM can optimize byte code execution, and JIT compilation can significantly improve performance by compiling frequently executed byte code into machine code.
Examples of JVM Languages:
Groovy: Primarily Interpreted
Groovy is primarily an interpreted language but can also be compiled using byte code and run on the JVM. This dual capability allows it to be both flexible and powerful, benefiting from the JVM's performance optimizations while maintaining the ease of an interpreted language.
Python: From Fully Interpreted to Hybrid Approach
Python originally started as a fully interpreted language. In its early versions, Python executed the source code directly, which was simple but not very efficient. To improve performance and maintainability, the Python development team introduced a hybrid approach combining compilation and interpretation elements.
How Python Uses Both Approaches:
- Compilation to Byte Code: When we run a Python script, Python compiles the source code into an intermediate form known as byte code. This byte code is a low-level representation of our code, optimized for execution by the Python Virtual Machine (PVM).
- Execution by the PVM: The Python Virtual Machine (PVM) interprets the byte code, executing it line by line. This allows Python to be both flexible and portable across different platforms.
By using byte code, Python combines the benefits of both compiled and interpreted languages:
- Portability: Byte code can run on any machine with a Python interpreter.
- Efficiency: Compiling to byte code improves performance over pure interpretation.
- Ease of Use: Python retains the flexibility and ease of debugging of an interpreted language.
With this understanding of compilers and interpreters, we’re ready to explore Python byte code in more detail. In the next section, we’ll dive into Python byte code, why it matters, and how it benefits Python development.
Transpilers
Transpilers, or source-to-source compilers, translate code from one high-level programming language to another. This is particularly common in languages that target JavaScript as a runtime environment. Transpilers enable developers to write code in more modern or feature-rich languages while still producing code that can run in environments where only JavaScript is supported.
Examples of Languages Using Transpilers:
- TypeScript: A superset of JavaScript that adds static types. TypeScript code is transpiled to JavaScript.
- Elm: A functional language for front-end development that compiles to JavaScript.
- CoffeeScript: A language that compiles JavaScript, aiming to enhance JavaScript's syntax.
Transpilers are mainly used when a project wants to piggyback on the popularity of tools from another programming language. For example, since Javascript was standardized and we can assume every browser will have a Javascript interpreter, any new language designed for web development will likely be transpiled to Javascript to gain the benefits from its obliquity and the speed of Google's V8 Javascript engine.
Another common scenario is when a newly compiled language doesn't want to create a full compiler that outputs machine code and instead compiles to a popular language like C, then C is compiled to the target matching language.
This could be for different reasons, like not having enough developers to create a full-blown language, such as a research project at a university.
What is Python Byte Code?
Before we explore the intricacies of Python byte code, let's first understand what byte code is and why it matters. Like many high-level programming languages, Python is designed to make programmers' lives easier. However, computers operate at a much lower level, understanding only machine code—a series of binary instructions.
When we write a Python script, we create human-readable high-level code. But for our computer to execute this script, it needs to translate it into a form it can understand. This is where Python byte code comes into play. Byte code is an intermediate representation of our Python code. It’s a low-level set of instructions that is more abstract than machine code but closer to what the computer understands than the original Python script.
Conclusion
As we have explored, the lines between compilers, interpreters, and transpilers are becoming progressively blurrier. Modern programming languages often adopt hybrid approaches that blend elements of both compilation and interpretation to balance performance, portability, and development ease.
To simplify:
- Interpreted Languages: We generally consider languages like Python or JavaScript to be interpreted because users receive the source code and run it directly on their computers using a program. These languages prioritize flexibility and ease of debugging, often translating and executing code line by line.
- Compiled Languages: Languages such as C, C++, and Rust are typically seen as compiled because users receive a non-human-readable format, such as a packed executable. These languages focus on performance, translating the entire program into machine code before execution. The binary the user receives is both OS and architecture-specific and if they have multiple machines with different OSes, they will need to download the program specific to each of them, provided the version for each of their machines exists.
- Intermediate Binary Formats: Languages like Java, which compile to an intermediate binary format (byte code), strike a balance between portability and efficiency. The users receive a binary package and won't see the source code like Python, but to execute the program, they need to have a virtual machine run it, such as the JVM or .Net. Virtual Machines can optimize performance further using Just-In-Time (JIT) compilation to maximize the performance in the specific machine they are running.
Understanding these differences helps us appreciate the trade-offs and design choices made by different programming languages. With this foundational knowledge, we're now well-equipped to delve deeper into Python byte code. Our next article explores Python byte code, why it matters, and how it benefits Python development.
Addendum: A Special Note for Our Readers
I decided to delay the introduction of subscriptions. You can read the full story here.
If you find our content helpful, there are several ways you can support us:
- The easiest way is to share our articles and links page on social media; it is free and helps us greatly.
- If you want a great experience during the Chinese New Year, I am renting my timeshare in Phuket. A five-night stay in this resort in Phuket costs 11,582 € on Expedia. I am offering it in USD at an over 40% discount compared to that price. I received the Year of the Snake in style.
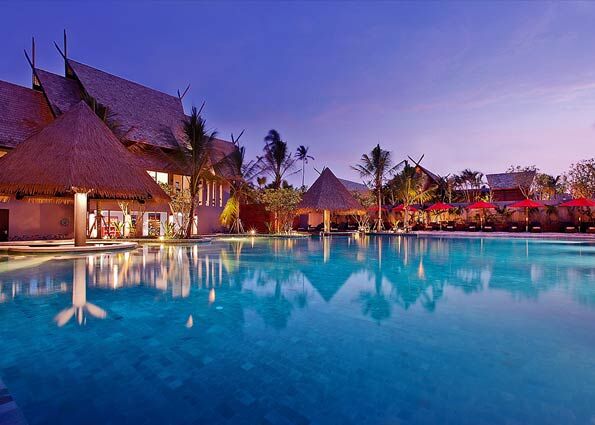
ReedWeek Timeshare Rental
- If your finances permit it, we are happy over any received donation. It helps us offset the site's running costs and an unexpected tax bill. Any amount is greatly appreciated:
- Finally, some articles have links to relevant goods and services; buying through them will not cost you more. And if you like programming swag, please visit the TuringTacoTales Store on Redbubble. Take a look. Maybe you can find something you like: